목록알고리즘 (10)
이야기박스
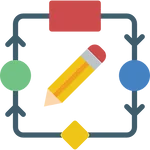
Problem Given a number, find the next smallest palindrome larger than this number. For example, if the input number is “2 3 5 4 5”, the output should be “2 3 6 3 2”. And if the input number is “9 9 9”, the output should be “1 0 0 1”. The input is assumed to be an array. Every entry in array represents a digit in input number. Let the array be ‘num[]’ and size of array be ‘n’ Example There can be..
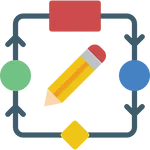
Problem Given a number n, print all primes smaller than or equal to n. It is also given that n is a small number. 정수 N이 주어집니다. 이 정수 N보다 작은 모든 소수를 찾으면 됩니다. Example Input : n =10 Output : 2 3 5 7 Input : n = 20 Output: 2 3 5 7 11 13 17 19 Approach 우선 소수에 대하여 다시 짚고 넘어가 보려 합니다. 소수란 자기 자신과 1 이외에는 다른 약수가 없는 수를 의미합니다. 그러면 우리는 어떤 수가 소수인지 아닌지 어떻게 알 수 있을까요? 모든 수에 대해서 한 번씩 나눠보면 될까요? 너무 비효율적인 방법입니다. 우리는 에라토..
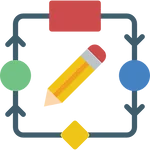
Problem Given a string str representing a number having N digits, the task is to calculate the number of ways to make the given number divisible by 3 by changing at most one digit of the number. 특정 정수 N이 주어집니다. 그리고 우리는 이 정수 N에서 하나의 자릿수의 숫자를 변경할 수 있습니다. 이때 변경할 수 있는 숫자 중, 3으로 나누어질 수 있는 숫자는 몇 개인지 확인해보는 문제입니다. Example 예시는 다음과 같습니다. Input: str[] = “23” Output: 7 Explanation: Below are the numbers tha..
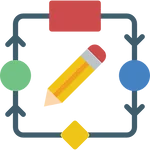
Problem Given a function random01Generator() that gives you randomly either 0 or 1, implement a function that utilizes this function and generate numbers between 0 and 6(both inclusive). All numbers should have same probabilities of occurrence. 먼저 0과 1을 생성하는 랜덤 함수가 주어집니다. 이제 우리는 위에 주어진 함수를 통하여 주어진 값 이하의 랜덤 숫자를 반환하는 함수를 만들어보면 됩니다. 주어진 문제에서는 6이라는 값이 Input으로 주어졌습니다. 하지만 우리는 6을 넘어서 INT_MAX 값까지 동작하는 ..
안녕하세요 !! 오늘은 정렬 알고리즘들을 많이 모아 정리해놨습니다 ㅎㅎ 목적은 각 알고리즘들이 수행시간 실험을 하기 위해서였습니다. 구성은 다음과 같습니다. ○ 일반 - 버블 정렬 (Bubble Sort) ○ 우선순위 큐 (Priority Queue) - 선택 정렬 (Selection Sort) - 삽입 정렬 (Insertion Sort) - 힙 정렬 (Heap Sort) ○ 분할 통치 기법 - 합병 정렬 (Merge Sort) - 퀵 정렬 (Quick Sort) ○ 기타 메소드 - checkTime() : 시간 측정 해줍니다 - swap() : 다들 아시죠? - print() : 리스트를 출력합니다 시험들이 끝나고 심심해서 막 달렸네요 ㅋㅋ 작년 이맘때 열심히 공부했었는데, 오랜만에 작성하려고 하니 책..
두 정렬 알고리즘 모두 Priority Queue의 개념을 이용합니다. § Priority Queue ADT란?데이터 항목이 자유롭게 삽입될 수 있는 저장소, 삭제는 키 값에 의해 결정된다.여기서 키란 가장 작은것 혹은 큰것 과 같이 기준을 제시해줌. 두 정렬을 요약하자면 아래와 같습니다.- 삽입 정렬은 리스트의 값이 자신의 위치를 찾아서 들어가는 것.- 선택 정렬은 주어진 위치에 알맞은 값을 찾아서 넣는 것. § 분석- 두 정렬 모두 O()의 전체적인 수행시간을 갖는다.- 기존의 리스트를 제외하면 O(1)의 추가 상수공간만 있으면 된다. § 간단한 C 구현 코드 #include #include #include #define SIZE100 void selection(int *arr); void inser..
후위수식 계산기입니다.마우스 클릭 혹은 키보드로 입력을 받고, 계산을 하는 방식입니다 ^^간단하게 정수의 계산만 구현했습니다. > Main - Frame을 키는 클래스Calculator - JPanel을 상속받아 전체적인 이벤트 및 화면을 담당하는 클래스NumPad - 버튼들을 담당하는 클래스History - 결과물을 출력해주는 클래스Convert - 후위 수식으로 전환해주는 클래스Evaluate - 후위 수식을 통해 계산을 해주는 클레스Stack - 스택 클래스 1. Main class import javax.swing.*; public class Main { public static void main(String[] args) { JFrame frame = new JFrame(); frame.setD..
자바로 하노이의 탑 UI를 만들었죠. 이제 그 근본이 된 코드. c로 짠 코드를 올리도록 하겠습니다. 이중재귀를 이용한 하노이의 탑입니다! #include <stdio.h> void move(int n, char from, char to) { // n번째 블록을 from에서 to로 옮겨라 printf("%dth %c %c\n", n, from, to); } int hanoi(int n,char from, char other, char to) { //printf("Checker >> n : %d, from : %c , other : %c, to : %c\n", n, from, other, to); if (n == 1) { move(n, from, to); return 0; } hanoi(n - 1,..